Introduction
Today there are a lot of applications and software systems that utilize remote databases to store specific information (for example, clinic’s patient data, online store user data, etc.).
To store information, both traditional relational databases and document-oriented databases can be used. Document-oriented databases are widely applied today for data storage thanks to their flexibility and ease of use. An example of such a database is Firebase Realtime Database. Data storage and exchange between the client application and the database occur using the popular JSON format.
When working with databases, you need to control users’ access to specific data within the database. Modern software systems support multi-user access to the database.
A user (for example, a customer of an online store) can sign up and gain the possibility to write or read specific data (for instance, it can be information about product orders, etc.). In this way, other users do not have access to the information of the current user and can work with their own data in the database without interfering with others.
Modern software systems also have support for user authentication, which is possible, for example, with the help of a username and password, a user’s mobile phone number, Google account, and so on.
In this article, we will consider the capabilities of the popular and feature-rich Firebase service for registering and authenticating a new user via the API. For user registration and authentication, we will use email.
We will demonstrate how to leverage Firebase Authentication capabilities in an Embarcadero Delphi FMX application with the help of the API. Additionally, we will implement the functionality for resetting a user’s password (in case a user forgets it).
The capabilities of the Firebase service for user registration and authentication are well-documented and presented in the form of the Firebase Auth REST API.
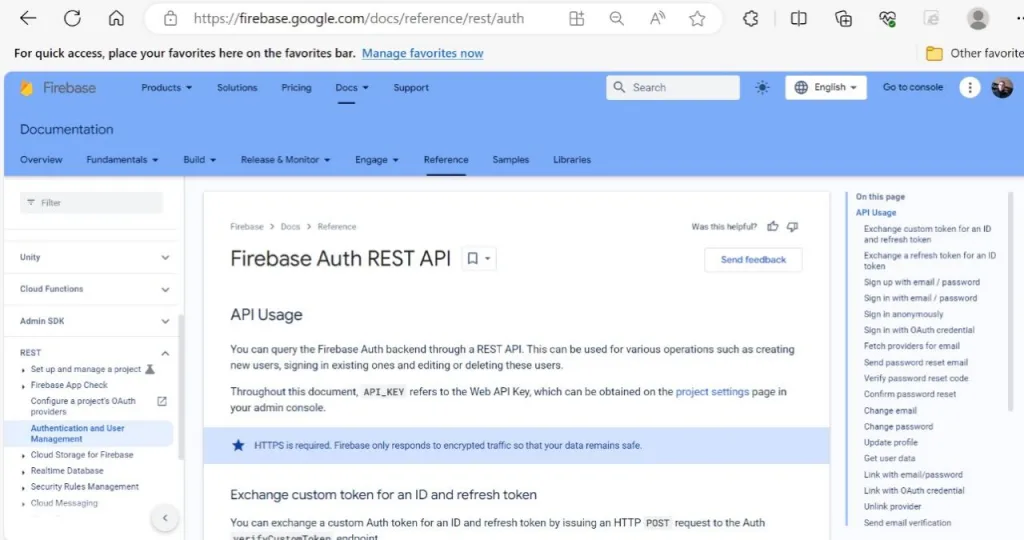
New Firebase project creation
To start using Firebase capabilities, you need to create a new Firebase project. To do this, go to the console.
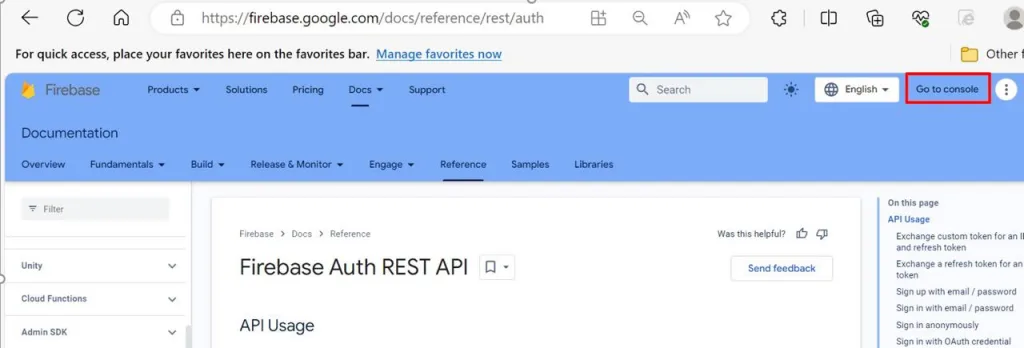
Now you should click on “Add project”
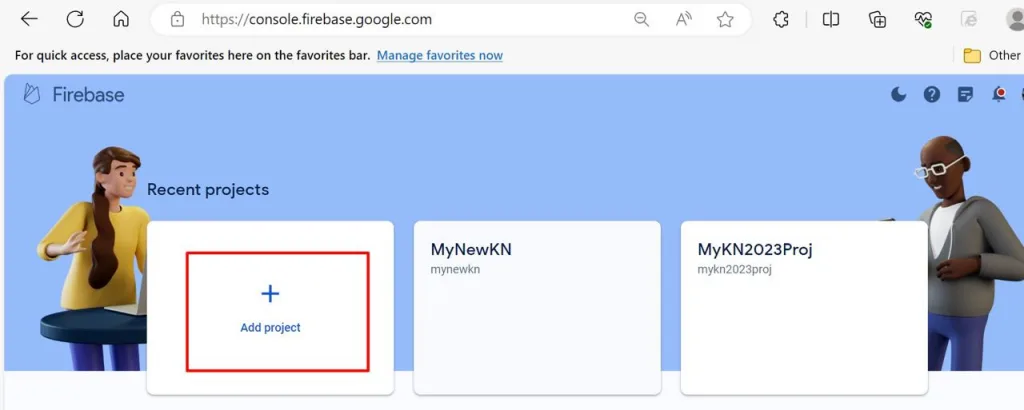
After that we will add a name for your project and press “Continue”.
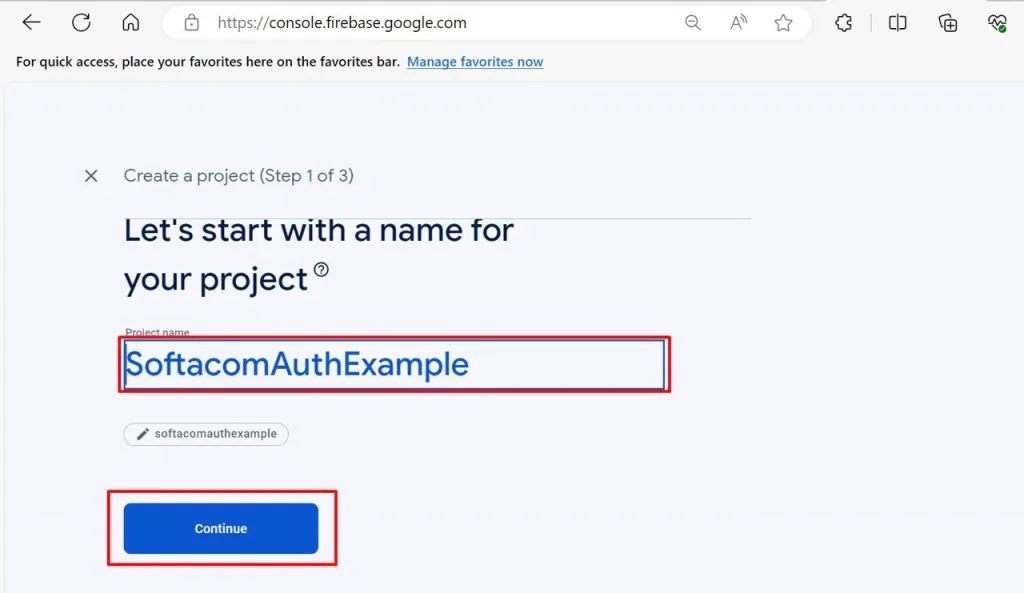
At the next step, you need to choose “Enable Google Analytics for this project” (optionally) and press “Continue”.

Now let’s choose “Default Account for Firebase” and click on “Create project”.
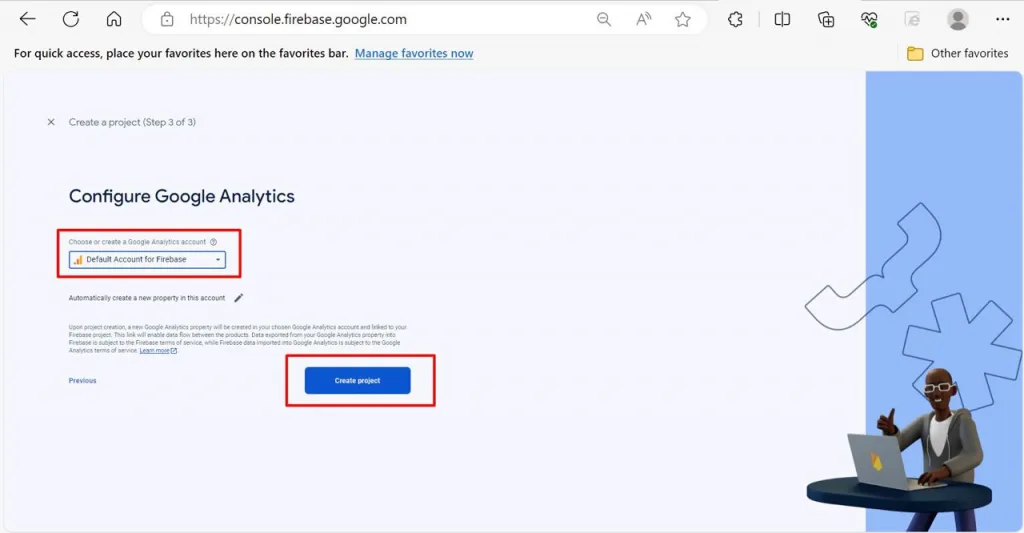
The process of the new project creation is starting.
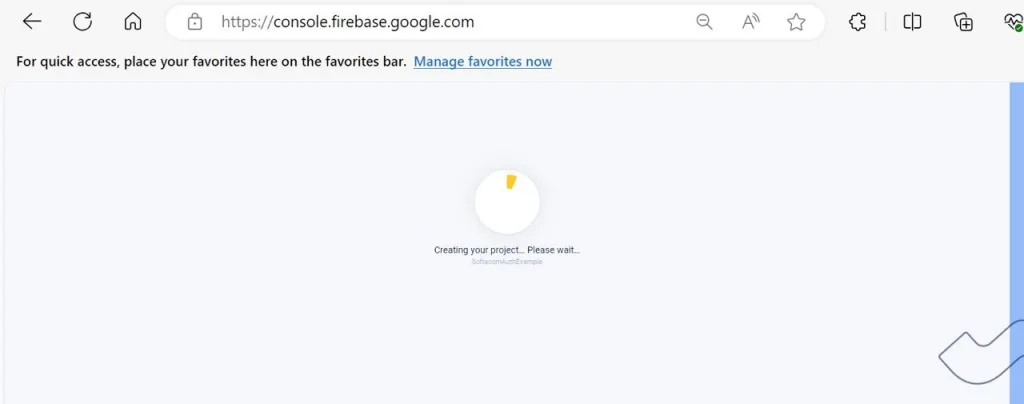
Then, you should click on “Continue”.
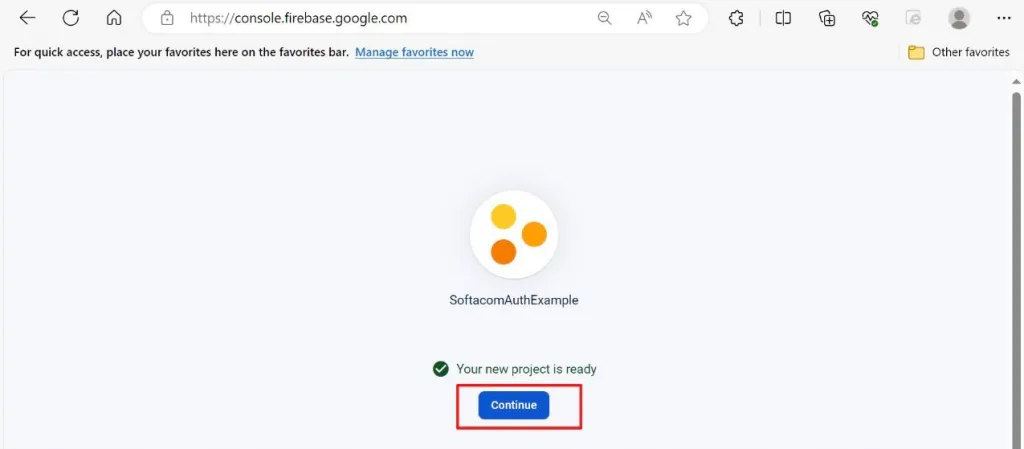
After completing all the above-mentioned operations the project will look the following way.
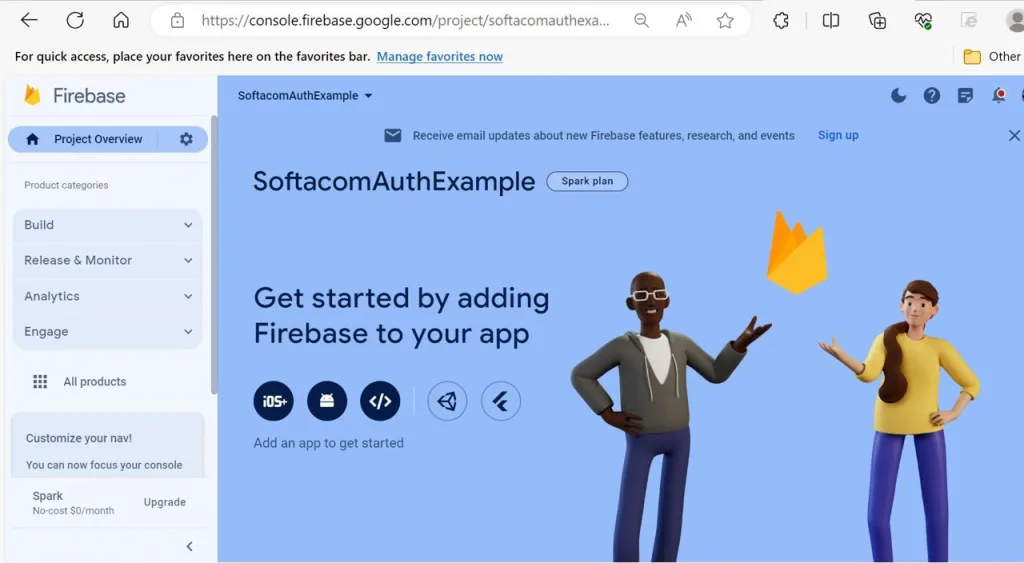
To enable the new user registration and authentication using email, let’s activate authentication in our Firebase project. To do this, go to the “Build” tab and select “Authentication”.
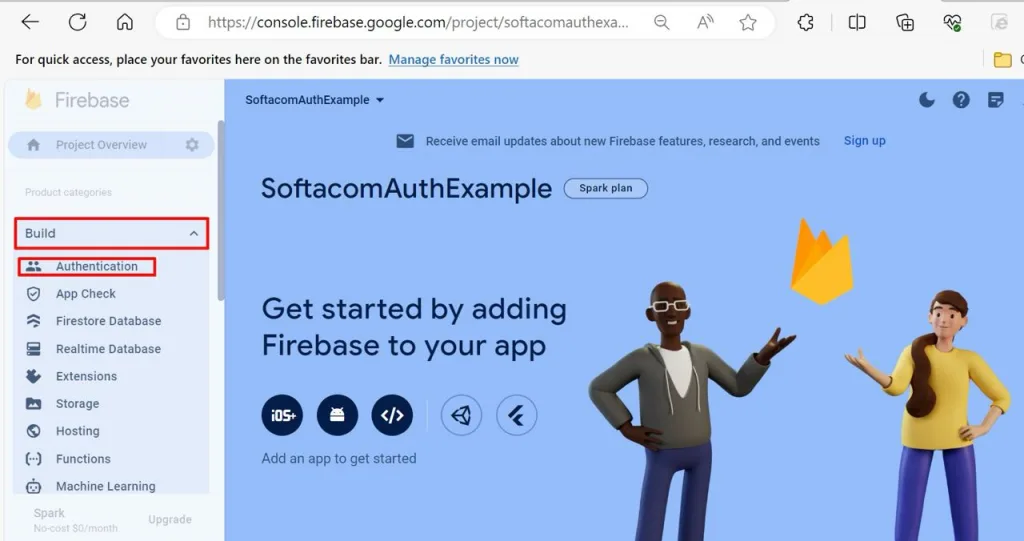
Then, let’s select “Get started”.

Now let’s choose “Email/Password”.
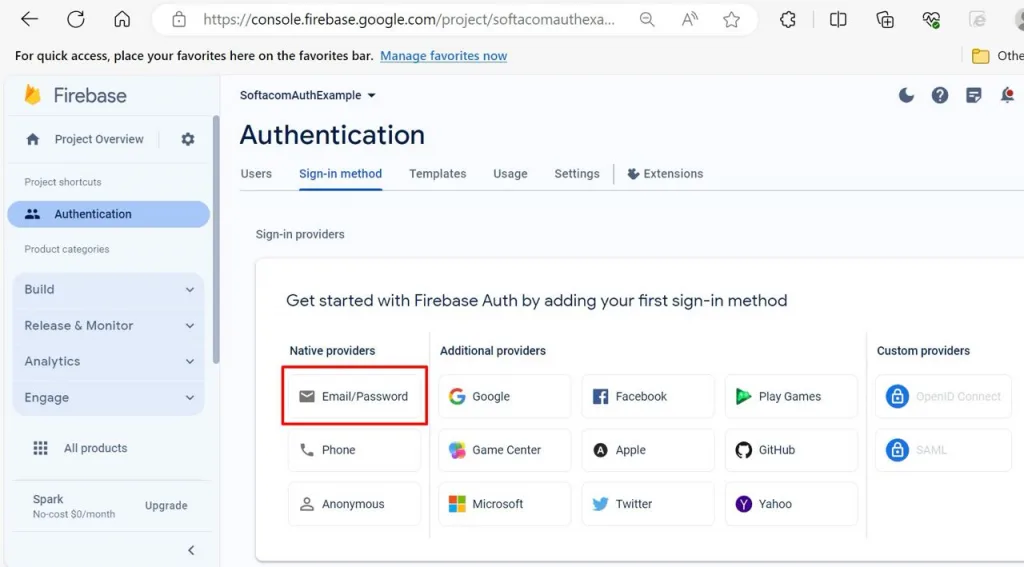
Now let’s select “Enable” and click on “Save”.

As a result, we have activated the Firebase service capability for registering and authenticating users with email.

To register and authenticate users via the API with subsequent integration into the Embarcadero Delphi FMX application, you need to obtain a Web API Key. To do this, choose “Project Settings”.

Then let’s choose Web API Key. To do it, we need to copy it and save it for its further use in our Delphi FMX app.
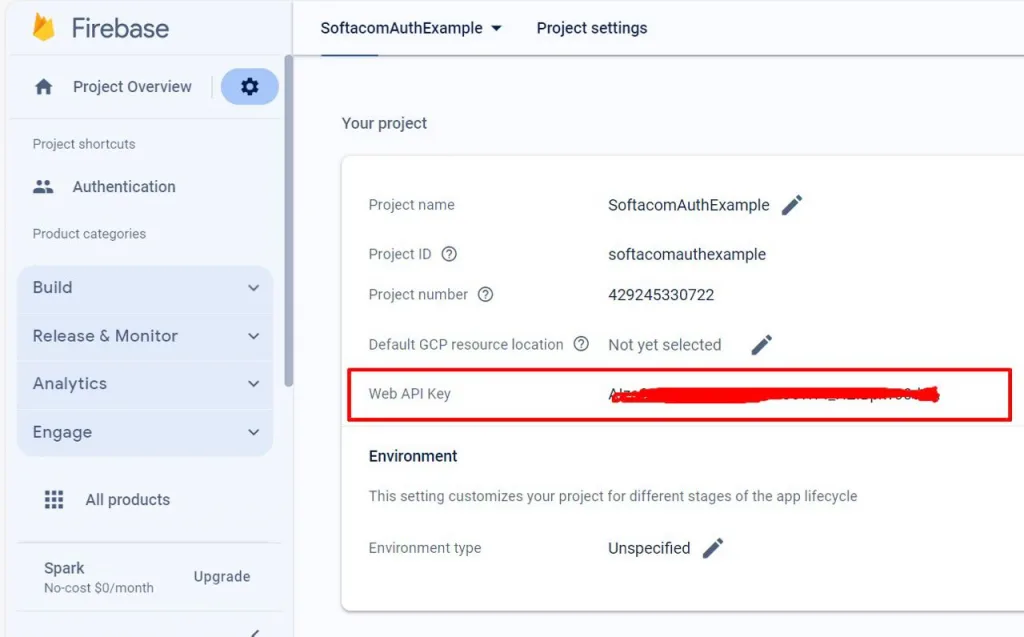
Description of the capabilities of the developed TFirebaseAuth class for user registration, authentication, and password reset
As an example, we will register and authenticate a new user and reset a password using our Delphi FMX application and Firebase API.
For the convenience of working with the Firebase API, we have developed a special class called TFirebaseAuth.
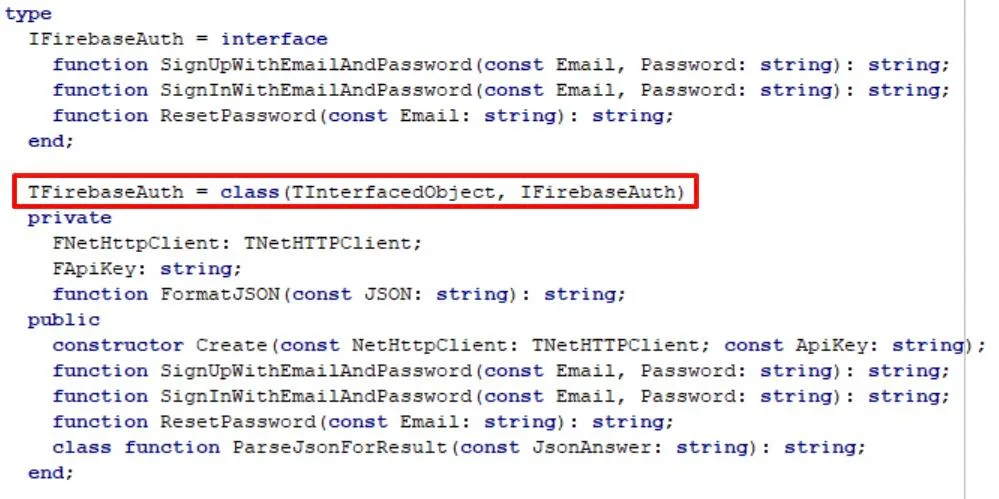
This class contains a constructor that takes an object of the TNetHttpClient class and a string constant “apikey,” which holds the Web API Key, as parameters.

The FormatJSON function allows formatting the response received from the Firebase service and presenting it to the user in a convenient and readable form.

The ParseJsonForResult method allows us to parse the JSON response from the Firebase service and return the result of user registration or authentication. In case of successful registration or authentication, a string value ‘email’ will be returned. In case of failure, ‘error’ will be returned.
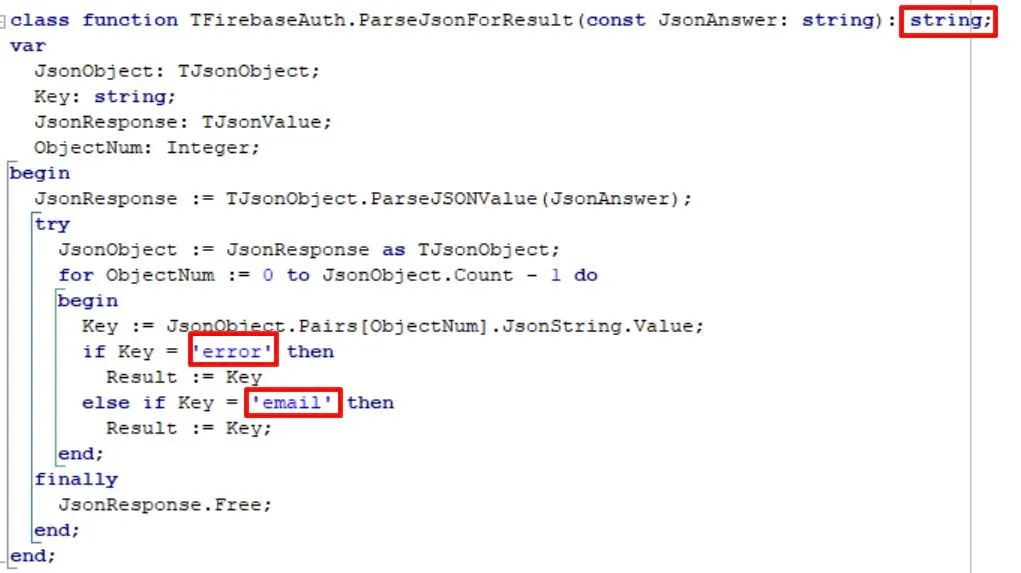
The SignUpWithEmailAndPassword method takes two input string parameters (email, password) and allows us to register a new user. User data, such as email and password, is transmitted using a POST request to the Firebase service API command URL.
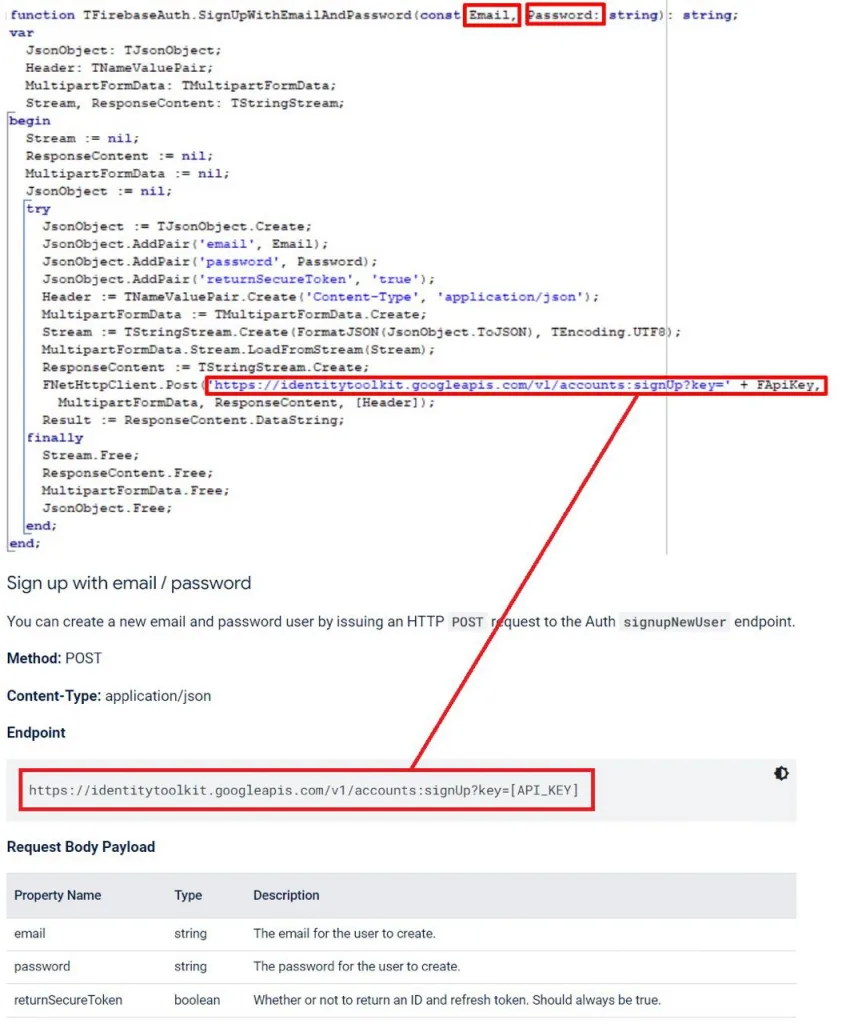
The SignInWithEmailAndPassword method takes two input string parameters (email, password) and performs user authentication. User data, such as email and password, is transmitted using a POST request to the Firebase service API command URL.
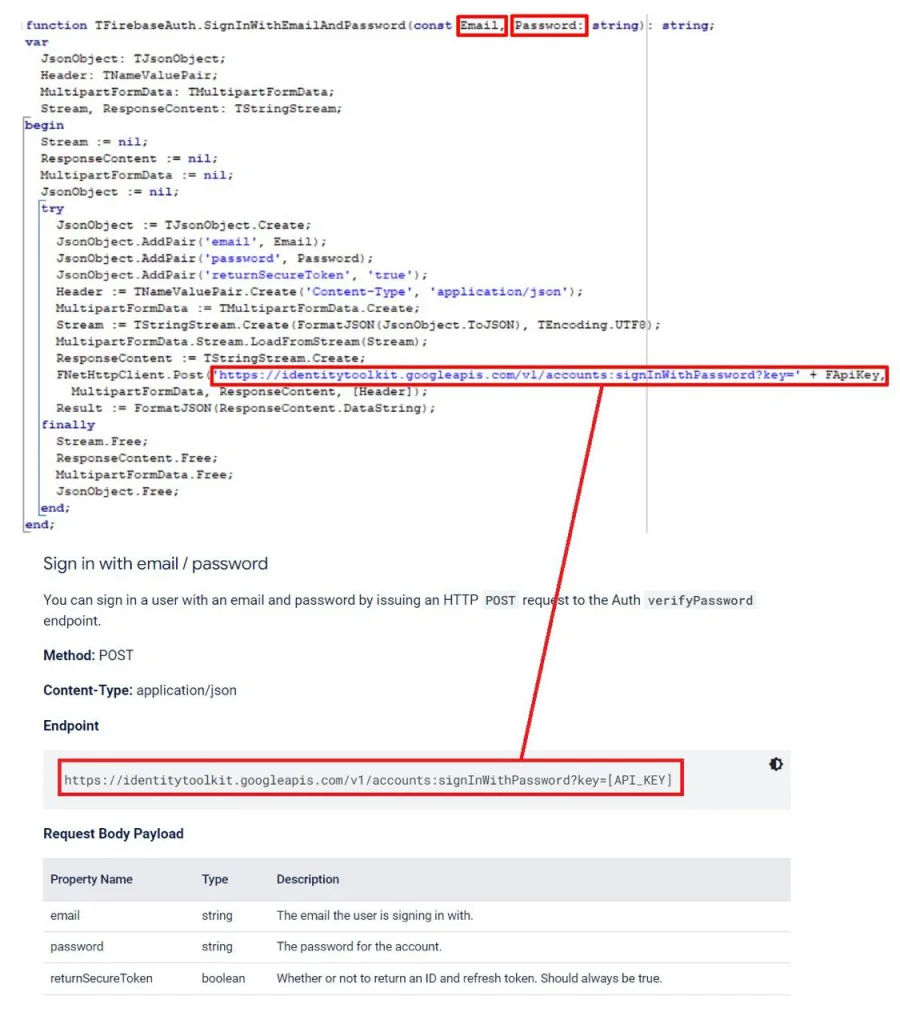
The ResetPassword method takes an input string parameter, email (user’s email), and performs a user password reset. The user receives an email where they can reset the forgotten password and create a new one. User data, such as email, is transmitted using a POST request to the Firebase service API command URL.
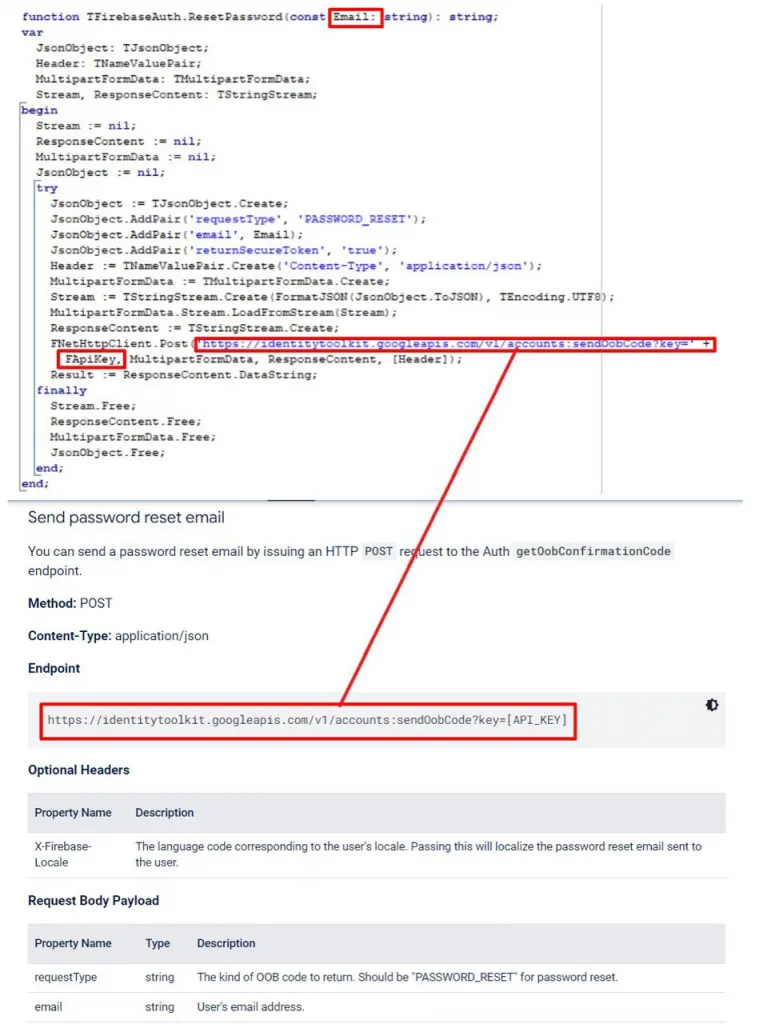
The source code for the TFirebaseAuth class is provided below
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384858687888990919293949596979899100101102103104105106107108109110111112113114115116117118119120121122123124125126127128129130131132133134135136137138139140141142143144145146147148149150151152153154155156157158159160161162163164165166167168169170171172173174175176177178179180 | unit FirebaseAuthClass;
interface
uses
System.SysUtils, System.Types, System.UITypes, System.Classes,
System.Variants, FMX.Types, FMX.Controls, FMX.Forms, FMX.Graphics,
FMX.Dialogs, FMX.Memo.Types, FMX.ScrollBox, FMX.Memo, FMX.StdCtrls,
FMX.Controls.Presentation, System.Net.URLClient, System.Net.HttpClient,
System.Net.HttpClientComponent, JSON, System.Threading,
System.Net.Mime, System.Generics.Collections;
type
IFirebaseAuth = interface
function SignUpWithEmailAndPassword(const Email, Password: string): string;
function SignInWithEmailAndPassword(const Email, Password: string): string;
function ResetPassword(const Email: string): string;
end;
TFirebaseAuth = class(TInterfacedObject, IFirebaseAuth)
private
FNetHttpClient: TNetHTTPClient;
FApiKey: string;
function FormatJSON(const JSON: string): string;
public
constructor Create(const NetHttpClient: TNetHTTPClient; const ApiKey: string);
function SignUpWithEmailAndPassword(const Email, Password: string): string;
function SignInWithEmailAndPassword(const Email, Password: string): string;
function ResetPassword(const Email: string): string;
class function ParseJsonForResult(const JsonAnswer: string): string;
end;
implementation
{ TFirebaseAuth }
constructor TFirebaseAuth.Create(const NetHttpClient: TNetHTTPClient; const ApiKey: string);
begin
FNetHttpClient := NetHttpClient;
if ApiKey <> '' then
FApiKey := ApiKey
else
begin
ShowMessage('Web API key is empty!');
Exit;
end;
end;
function TFirebaseAuth.FormatJSON(const JSON: string): string;
var
JsonObject: TJsonObject;
begin
JsonObject := TJsonObject.ParseJSONValue(JSON) as TJsonObject;
try
Result := JsonObject.Format();
finally
JsonObject.Free;
end;
end;
class function TFirebaseAuth.ParseJsonForResult(const JsonAnswer: string): string;
var
JsonObject: TJsonObject;
Key: string;
JsonResponse: TJsonValue;
ObjectNum: Integer;
begin
JsonResponse := TJsonObject.ParseJSONValue(JsonAnswer);
try
JsonObject := JsonResponse as TJsonObject;
for ObjectNum := 0 to JsonObject.Count – 1 do
begin
Key := JsonObject.Pairs[ObjectNum].JsonString.Value;
if Key = 'error' then
Result := Key
else if Key = 'email' then
Result := Key;
end;
finally
JsonResponse.Free;
end;
end;
function TFirebaseAuth.ResetPassword(const Email: string): string;
var
JsonObject: TJsonObject;
Header: TNameValuePair;
MultipartFormData: TMultipartFormData;
Stream, ResponseContent: TStringStream;
begin
Stream := nil;
ResponseContent := nil;
MultipartFormData := nil;
JsonObject := nil;
try
JsonObject := TJsonObject.Create;
JsonObject.AddPair('requestType', 'PASSWORD_RESET');
JsonObject.AddPair('email', Email);
JsonObject.AddPair('returnSecureToken', 'true');
Header := TNameValuePair.Create('Content-Type', 'application/json');
MultipartFormData := TMultipartFormData.Create;
Stream := TStringStream.Create(FormatJSON(JsonObject.ToJSON), TEncoding.UTF8);
MultipartFormData.Stream.LoadFromStream(Stream);
ResponseContent := TStringStream.Create;
FNetHttpClient.Post('https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode?key=' +
FApiKey, MultipartFormData, ResponseContent, [Header]);
Result := ResponseContent.DataString;
finally
Stream.Free;
ResponseContent.Free;
MultipartFormData.Free;
JsonObject.Free;
end;
end;
function TFirebaseAuth.SignInWithEmailAndPassword(const Email, Password: string): string;
var
JsonObject: TJsonObject;
Header: TNameValuePair;
MultipartFormData: TMultipartFormData;
Stream, ResponseContent: TStringStream;
begin
Stream := nil;
ResponseContent := nil;
MultipartFormData := nil;
JsonObject := nil;
try
JsonObject := TJsonObject.Create;
JsonObject.AddPair('email', Email);
JsonObject.AddPair('password', Password);
JsonObject.AddPair('returnSecureToken', 'true');
Header := TNameValuePair.Create('Content-Type', 'application/json');
MultipartFormData := TMultipartFormData.Create;
Stream := TStringStream.Create(FormatJSON(JsonObject.ToJSON), TEncoding.UTF8);
MultipartFormData.Stream.LoadFromStream(Stream);
ResponseContent := TStringStream.Create;
FNetHttpClient.Post('https://identitytoolkit.googleapis.com/v1/accounts:signInWithPassword?key=' + FApiKey,
MultipartFormData, ResponseContent, [Header]);
Result := FormatJSON(ResponseContent.DataString);
finally
Stream.Free;
ResponseContent.Free;
MultipartFormData.Free;
JsonObject.Free;
end;
end;
function TFirebaseAuth.SignUpWithEmailAndPassword(const Email, Password: string): string;
var
JsonObject: TJsonObject;
Header: TNameValuePair;
MultipartFormData: TMultipartFormData;
Stream, ResponseContent: TStringStream;
begin
Stream := nil;
ResponseContent := nil;
MultipartFormData := nil;
JsonObject := nil;
try
JsonObject := TJsonObject.Create;
JsonObject.AddPair('email', Email);
JsonObject.AddPair('password', Password);
JsonObject.AddPair('returnSecureToken', 'true');
Header := TNameValuePair.Create('Content-Type', 'application/json');
MultipartFormData := TMultipartFormData.Create;
Stream := TStringStream.Create(FormatJSON(JsonObject.ToJSON), TEncoding.UTF8);
MultipartFormData.Stream.LoadFromStream(Stream);
ResponseContent := TStringStream.Create;
FNetHttpClient.Post('https://identitytoolkit.googleapis.com/v1/accounts:signUp?key=' + FApiKey,
MultipartFormData, ResponseContent, [Header]);
Result := ResponseContent.DataString;
finally
Stream.Free;
ResponseContent.Free;
MultipartFormData.Free;
JsonObject.Free;
end;
end;
end. |
Implementation of user registration, authentication, and password reset features in our FMX Delphi application
For interacting with the Firebase API, and specifically for sending POST requests to the Firebase service in our Delphi FMX application, we will use the TNetHttpClient component.
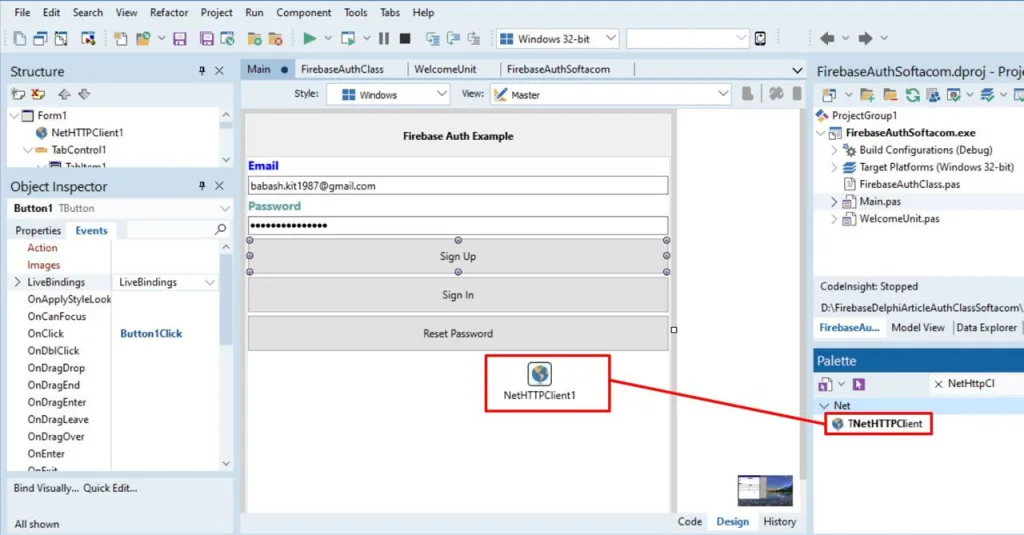
This component does not require additional settings. It is enough to simply drag it onto the form from the component palette (Net tab).
The user’s email and password in the application are entered using components of the TEdit class.

To display the JSON response from the Firebase service in a Delphi FMX application, we will use the standard TMemo component (Standard tab).

The capabilities for user registration, authentication, and password reset are implemented in button handlers (TButton class from the Standard tab of the component palette).

Let’s consider the implementation of user registration with the help of the Firebase API with our Delphi FMX application.
In the button Sign Up event handler, we will declare an object of the IFirebaseAuth type to work with the Firebase service, and a string variable JsonAnswer to store the JSON response from the service.

Now it is necessary to call the constructor of the TFirebaseAuth class and pass our NetHttpClient1 object of the TNetHttpClient class and the FWebApiKey field to it.
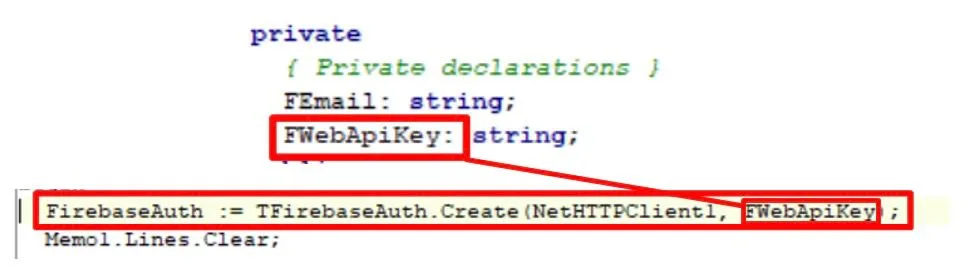
The field FWebApiKey contains our pre-saved Web API Key from the Firebase project. In the OnCreate method of the main form, we should assign the Web API Key value from the Firebase project to the FWebApiKey field.
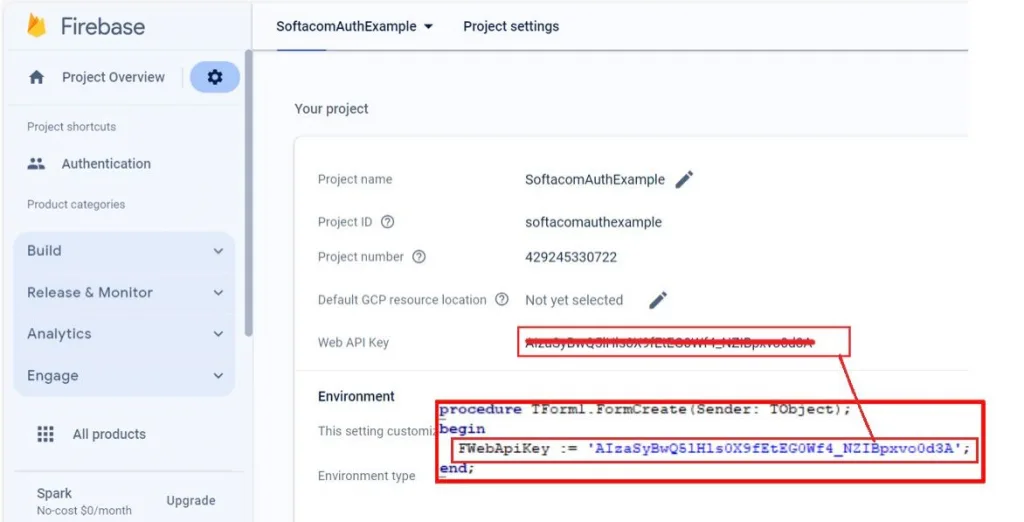
For the correct operation of the application during the server request, we will use the TTask class and its Run method. This will allow us to make a POST request in a parallel thread, preventing the application from hanging during operation. Additionally, we will call the SignUpWithEmailAndPassword method, passing the string values of the TEdit class fields (email and password) to it.

To update the state of components, specifically to assign the received response from the Firebase service in JSON (string variable JsonAnswer, which stores the response from the Firebase service) to the Text property of the multiline input field Memo1 of the TMemo class, we will use the TThread.Synchronize method.
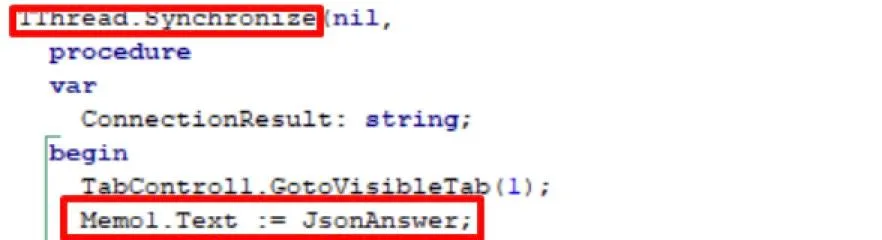
To obtain information about the success of the registration, we will use the ParseJsonForResult method. This method takes the JSON response from the Firebase service as a parameter. The method returns the string ‘email’ in case of success and ‘error’ in case of an error.

The source code of the Sign Up button handler is provided below
1234567891011121314151617181920212223242526 | procedure TForm1.Button1Click(Sender: TObject);
var
FirebaseAuth: IFirebaseAuth;
JsonAnswer: string;
begin
FirebaseAuth := TFirebaseAuth.Create(NetHTTPClient1, FWebApiKey);
Memo1.Lines.Clear;
TTask.Run(
procedure
begin
JsonAnswer := FirebaseAuth.SignUpWithEmailAndPassword(EditEmail.Text, EditPassword.Text);
TThread.Synchronize(nil,
procedure
var
ConnectionResult: string;
begin
TabControl1.GotoVisibleTab(1);
Memo1.Text := JsonAnswer;
ConnectionResult := TFirebaseAuth.ParseJsonForResult(JsonAnswer);
if ConnectionResult = 'error' then
ShowMessage('Error! Wrong password or login or account created!')
else if ConnectionResult = 'email' then
ShowMessage('Account created!');
end);
end);
end; |
Let us demonstrate how it is possible to register a new user in our Delphi FMX application.

In case the account already exists, the application will display an error message.

Let’s consider the possibilities of user authentication using the Firebase API in our application. Upon successful authentication, we will display a welcome form to the user, including email. To achieve this, it is necessary to call the Form2 form from the main form Form1 using the Form2.Show method (TForm class). For user authentication, we use the SignUpWithEmailAndPassword method of the TFirebaseAuth class, passing email and password as parameters. In case of an incorrect password or login, an error message will be displayed to the user. Otherwise, the implementation is similar to user registration.
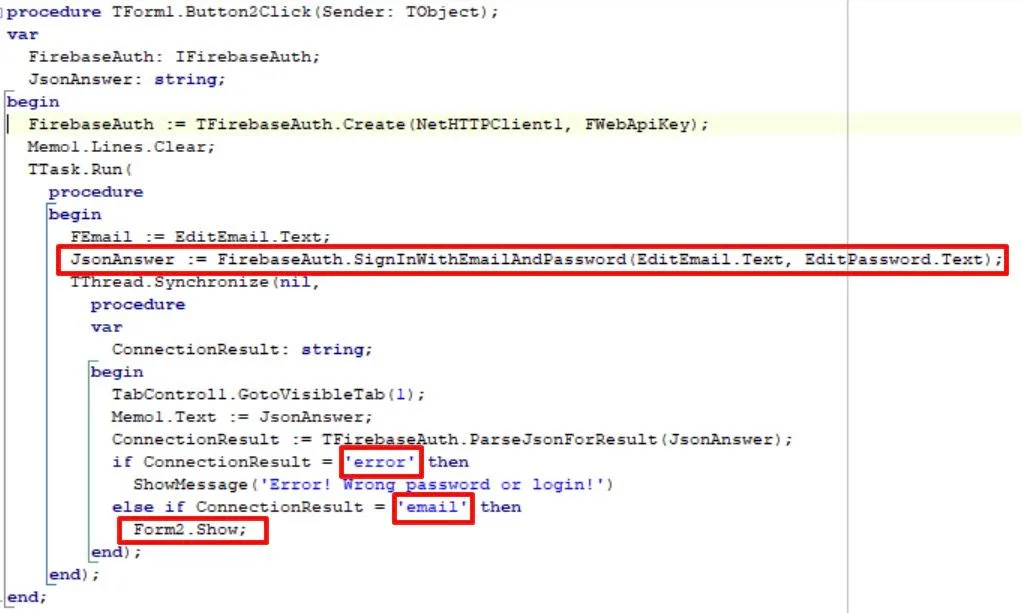
The source code of the Sign In button handler is provided below
123456789101112131415161718192021222324252627 | procedure TForm1.Button2Click(Sender: TObject);
var
FirebaseAuth: IFirebaseAuth;
JsonAnswer: string;
begin
FirebaseAuth := TFirebaseAuth.Create(NetHTTPClient1, FWebApiKey);
Memo1.Lines.Clear;
TTask.Run(
procedure
begin
FEmail := EditEmail.Text;
JsonAnswer := FirebaseAuth.SignInWithEmailAndPassword(EditEmail.Text, EditPassword.Text);
TThread.Synchronize(nil,
procedure
var
ConnectionResult: string;
begin
TabControl1.GotoVisibleTab(1);
Memo1.Text := JsonAnswer;
ConnectionResult := TFirebaseAuth.ParseJsonForResult(JsonAnswer);
if ConnectionResult = 'error' then
ShowMessage('Error! Wrong password or login!')
else if ConnectionResult = 'email' then
Form2.Show;
end);
end);
end; |
When displaying Form2, set the Text property of the Label1 component of the TLabel class to the user’s email.

Let’s demonstrate the user authentication functionality in our Delphi FMX application.
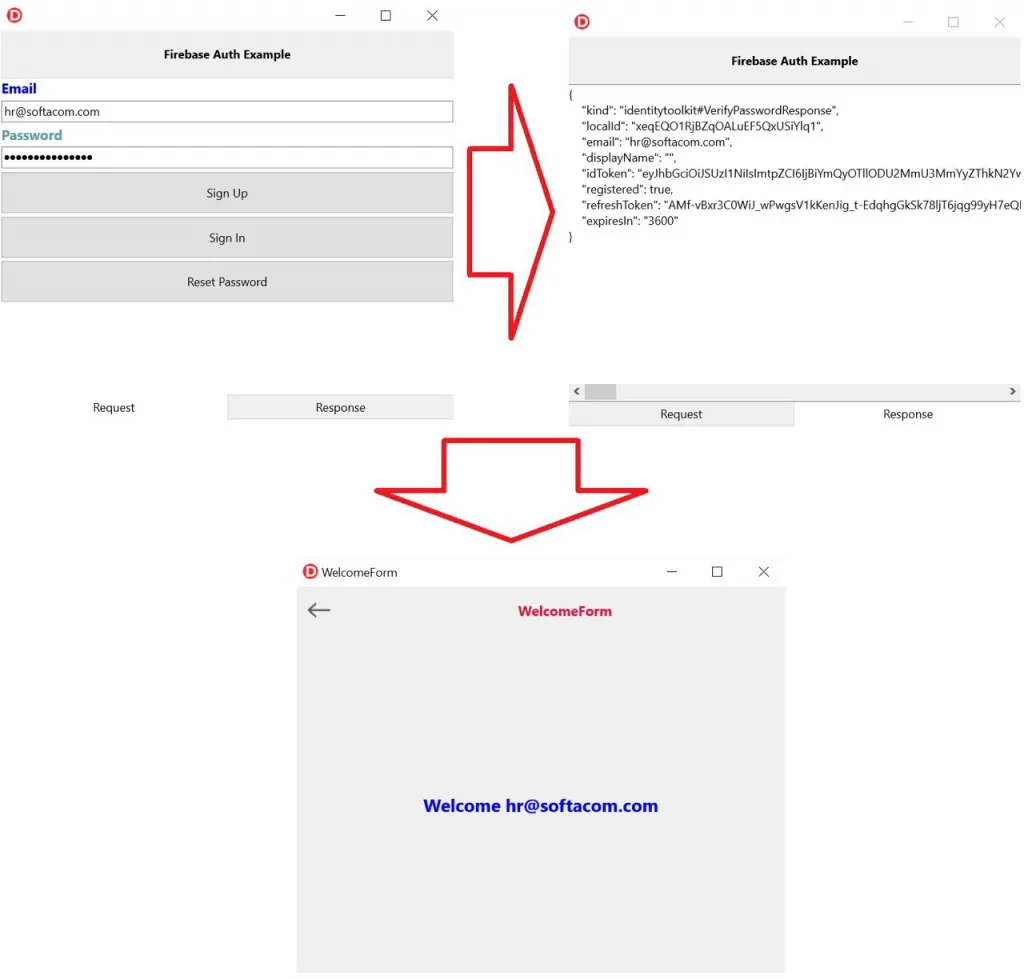
In case of an incorrectly entered login (email) or password, we will receive an error message.
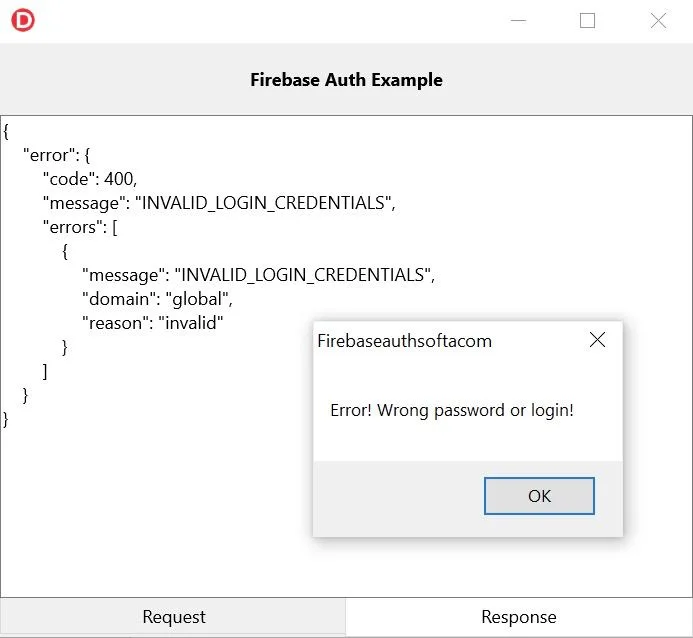
Let’s consider the option of resetting a user’s password using the Firebase API in our application. To do this, we will use the ResetPassword method of the TFireBaseAuth class. We shouldpass the email as a parameter to this method.

The source code for the Reset Password button handler is shown below
12345678910111213141516171819 | procedure TForm1.Button3Click(Sender: TObject);
var
FirebaseAuth: IFirebaseAuth;
JsonAnswer: string;
begin
FirebaseAuth := TFirebaseAuth.Create(NetHTTPClient1, FWebApiKey);
Memo1.Lines.Clear;
TTask.Run(
procedure
begin
TabControl1.GotoVisibleTab(1);
JsonAnswer := FirebaseAuth.ResetPassword(EditEmail.Text);
TThread.Synchronize(nil,
procedure
begin
Memo1.Text := JsonAnswer;
end);
end);
end; |
Let’s demonstrate in our Delphi FMX application the use of the password reset feature for the user.
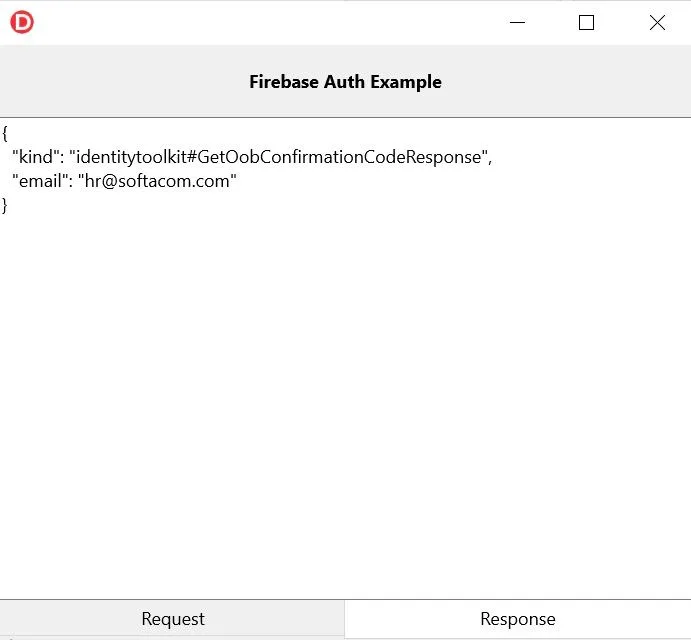
As a result, a message will be sent to the user’s email address, allowing us to change the forgotten password.
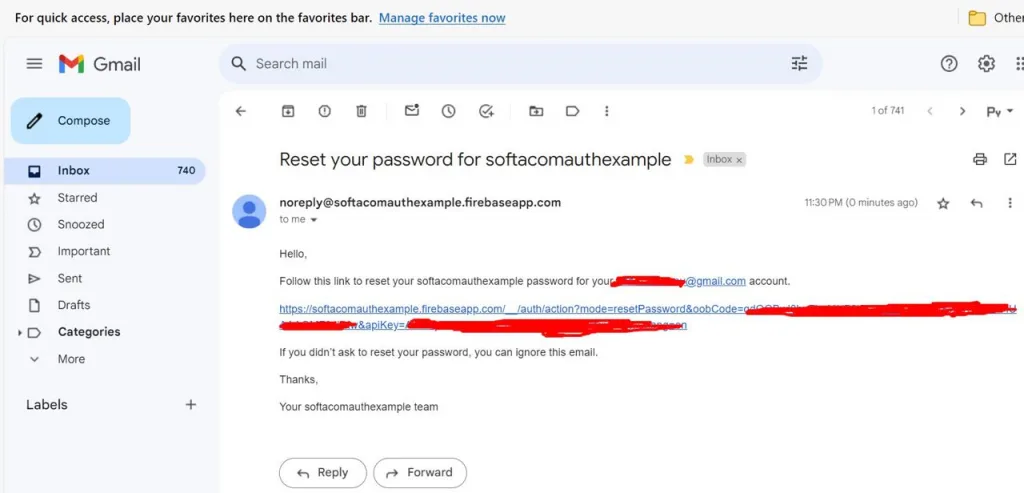
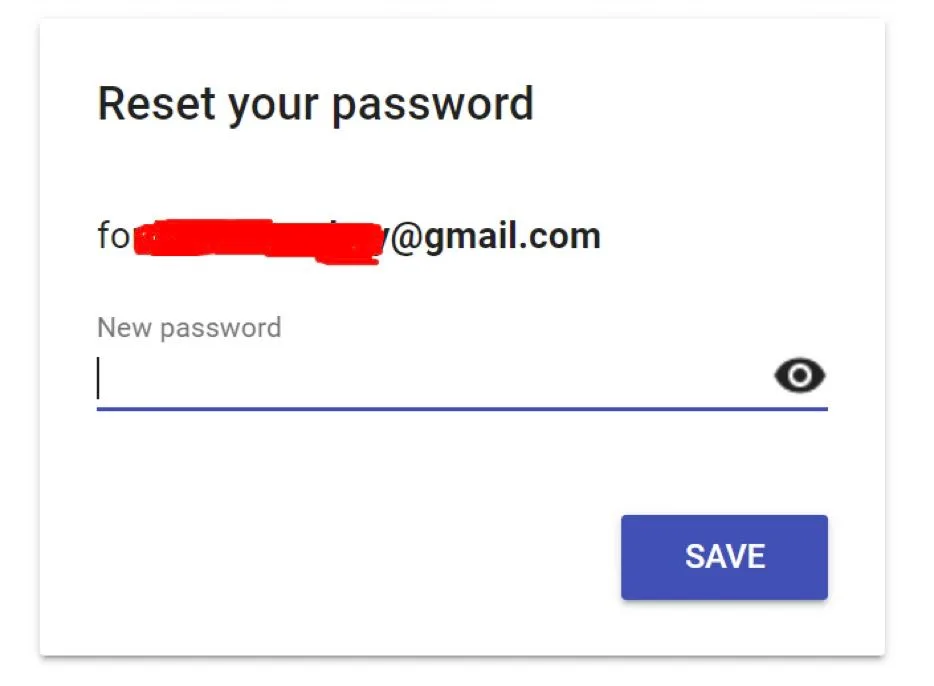
Check out our video on how to work with the API of various REST services